Manual API Testing with Postman – A Beginner-Friendly Guide
Beginner's guide to manual API testing using Postman. The post Manual API Testing with Postman – A Beginner-Friendly Guide appeared first on Spritle software.
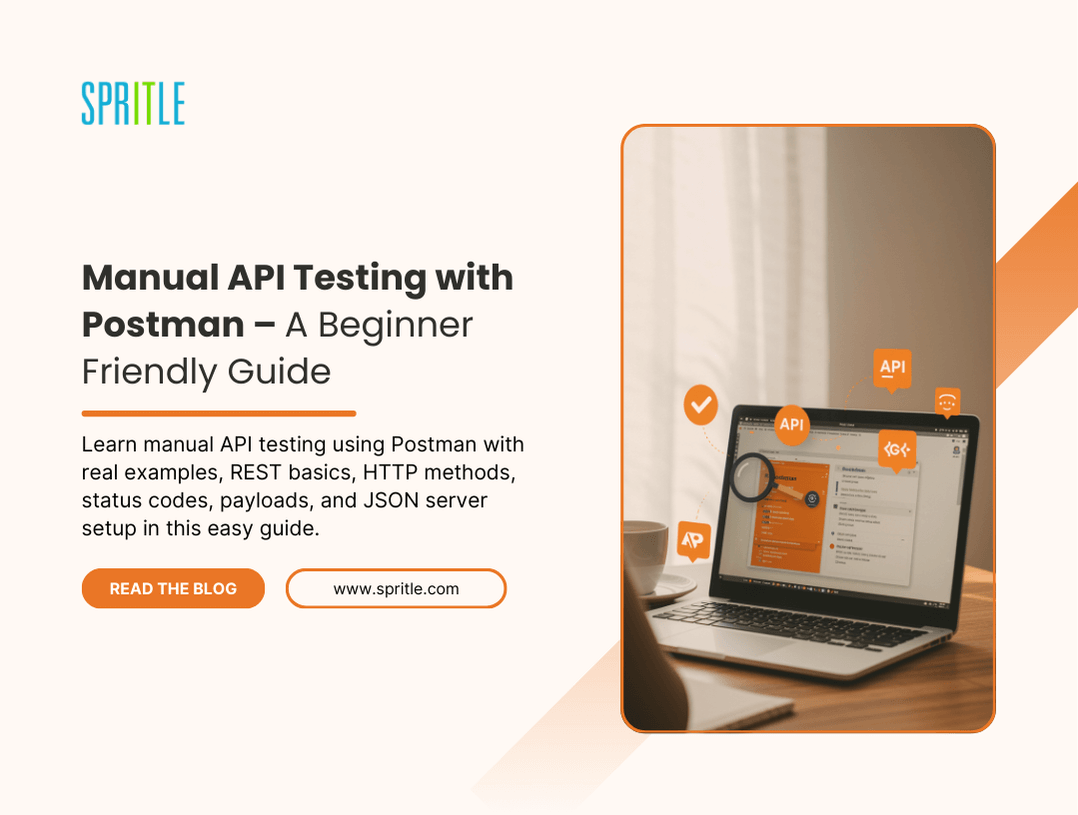
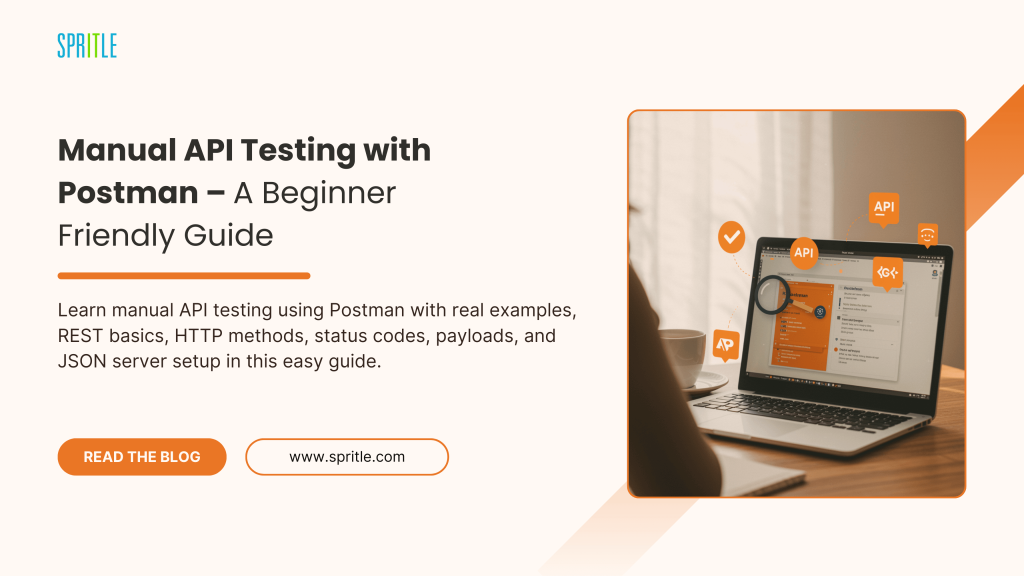
Introduction
APIs, or Application Programming Interfaces, are the invisible connectors that let different software systems talk to each other.They make it possible for apps to share data, perform actions, and deliver seamless user experiences.Whether you’re ordering food or checking maps, APIs are working behind the scenes.In this blog, we’ll explore what APIs are, how they work, and why they matter.By the end, you’ll have a clear understanding of how APIs power the apps we use every day.
What is an API ?
- API expands as Application Programming Interface.
- API (Application Programming Interface) is a set of rules and protocols that allow different software applications to communicate with each other. It acts as an intermediary, enabling one program to request data or services from another.
- It is the way of communication between two applications where apps may differ in their platforms or in terms of technology.
For instance: Front-end of the app -> middle layer (API) -> Back-end of the app
The middle layer is used for both fetching data from the backend and inserting front-end data into the backend.
EXAMPLE: Imagine you order food on Swiggy or Zomato, and you can see the delivery partner moving on the map. Google Maps API acts like a helper, giving Swiggy/Zomato all the location and route details they need to keep you updated.
Types of API :
We can categorize APIs into many categories, but in most cases, APIs are categorized into two types: SOAP and REST.
- SOAP (Simple Object Access Protocol) -> supports only XML data
- REST (Representational State Transfer) -> supports many formats like XML, JSON, HTML
Both are web services. (An API on the internet is called a web service, and all web services are APIs.)
A web service is an API wrapped in HTTP. A web service needs a network, while an API doesn’t need a network for its operation.
Understanding REST API
- REST API (Representational State Transfer API) is a type of web API that follows the principles of REST, an architectural style for designing networked applications.
- REST APIs use standard HTTP methods to allow communication between a client (e.g., your browser or app) and a server.
- HTTP methods are (GET, POST, PUT, DELETE). We can also identify it as CRUD operations (Create, Read, Update, Delete).
This is how REST APIs work: Request Message -> API -> Response Message.
Example: While we are searching for hotels on some website, it is the GET method; ordering food is the POST method; changing the food is the PUT method; and cancelling the order is the DELETE method.
Client vs Server: What’s the Difference?
- A client is any device, software, or application that makes requests to a server to access or interact with resources or services. A client can be a browser or hardware device that accesses a service made by a server.
The client is typically the user-facing component in a client-server architecture.
- A server is a powerful computer or software system that provides services, resources, or data to other computers or clients over a network.
It handles requests from clients (such as browsers or apps), processes them, and sends back the required response or data.
Client -> Internet -> Server
CLIENT / SERVER ARCHITECTURE :
Client-Server Architecture is a model used in networking where two distinct entities, the client and the server, interact to provide services or resources.
The architecture is categorized as One-Tier Architecture, Two-Tier Architecture, Three-Tier Architecture, and N-Tier Architecture.
One-Tier Architecture (Single-Tier):
- The client and the server are on the same machine or in a very simple setup where the client accesses a local server.
- (Example: A local database application.)
Two-Tier Architecture:
- The client interacts directly with the server. This is the most common model.
- (Example: A web browser interacting with a web server.)
Three-Tier Architecture:
- The architecture is divided into three layers: Presentation Layer (Client), Application Layer (Server), and Database Layer (Data Storage). The client communicates with the application server, which in turn communicates with the database server.
Example: A web application where the client interacts with a web server, and the server communicates with a database to fetch data.
Presentation Layer (HTML, CSS, JS) → Application Layer (Java, Python, C++, C#) → Data Layer (MySQL, PostgreSQL, MongoDB)
N-Tier Architecture:
An extension of the three-tier model, where there can be multiple intermediate layers for handling specific tasks (e.g., a separate authentication server, business logic server, etc.).
(Example: Large-scale enterprise applications with multiple specialized servers
Understanding API Terminologies: URI, URL, and URN
The basic terminologies we need to know about are URI, URL, and URN.
URI (Uniform Resource Identifier):
A URI is a unique string of characters used to identify a resource on the internet or within a system.
It provides a way to reference or locate a resource, whether it’s a webpage, image, file, or any other type of data.
Example: “http://google.com/articles/articlename” – Google.com alone is the URI (just an identifier of the domain).
URL (Uniform Resource Locator):
A URL is a specific type of URI that not only identifies a resource but also provides the means to locate it on the internet.
It includes both the address of the resource and the protocol used to access it.
Example: “http://google.com/articles/articlename” – The whole URL (full address with protocol and domain).
URN (Uniform Resource Name):
A URN is a specific type of URI that uniquely identifies a resource by name within a particular namespace, without providing the location or method of accessing that resource.
Unlike a URL, a URN does not include the address or protocol to access the resource. Instead, it provides a unique identifier for the resource.
Example: “http://google.com/articles/articlename” – articles/articlename is the URN, also known as the endpoint.
Features and Resources in APIs
Feature:
A feature generally refers to a specific characteristic, functionality, or capability of a system, application, or service.
Feature is the term used in manual testing to test some functionality.
Features are aspects that provide value to the user and define the system’s behavior or what it can do.
Example: In a messaging app, features might include sending messages, creating groups, audio calls, and file sharing.
Resource:
A resource refers to any entity or object that can be accessed, manipulated, or interacted with within a system. In the context of APIs or web services, a resource typically refers to data or objects that are made available through an API and can be created, read, updated, or deleted.
Example: Product could be a resource accessible at: https://api.example.com/products
Payload in APIs
- In the context of APIs and web development, payload refers to the data that is sent with an HTTP request or response.
- It is the actual content or message being transmitted from the client to the server (or vice versa), which is processed or used by the server or client.
Payload typically refers to the body of the HTTP request or response .
TYPES OF PAYLOAD
It is mainly categorized into two types such as Request Payload and Response Payload.
1.Request Payload: The data you send in the body of a request (e.g., when creating or updating a resource).
Example: Sending JSON data in a POST request to create one user:
jsonCopyEdit{
"username": "johndoe",
"email": "john@example.com"
}
2.Response Payload: The data sent back in the body of the response (e.g., when fetching data or confirmation of an action).
Example: Response Payload of previously created user:
jsonCopyEdit{
"status": "success",
"message": "User created successfully"
}
Getting Started with Postman
- Postman is a popular API testing and development tool that allows users to send requests (like GET, POST, PUT, DELETE) to APIs and view the responses.
We can do the following things in Postman:
- Creating and managing collections of API requests.
- Sending requests with custom headers, parameters, and body data.
- Viewing responses in different formats (JSON, XML, etc.).
- Automating tests with pre-defined scripts.
- After installing Postman, there will be an option named ‘Workspace’. A workspace is an area where we maintain files and save them.
- It will be saved to our logged-in Gmail ID.
Try to create one workspace, and while creating it, we need to select which type we want and go with the API Testing option.
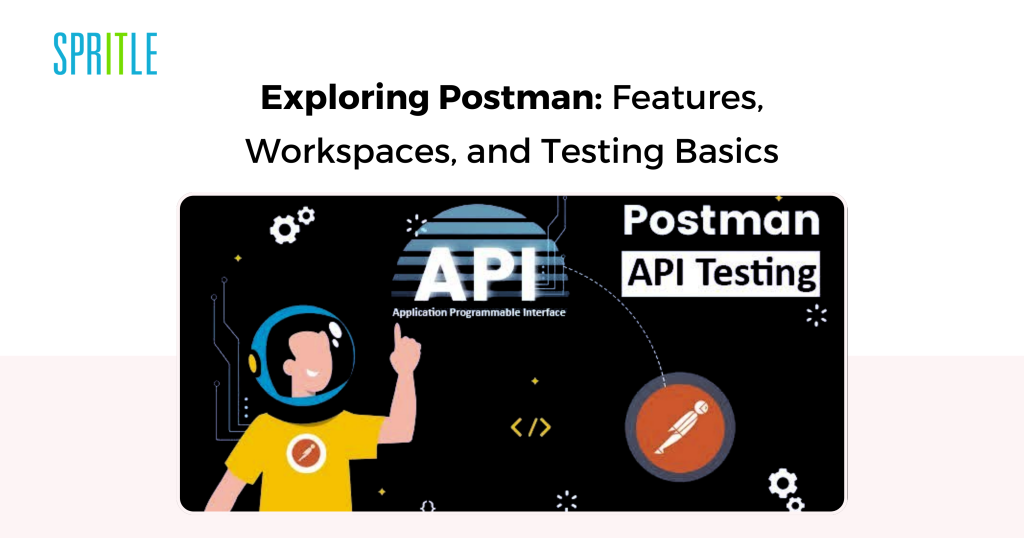
Collections in Postman
- In Postman, a collection is a group of API requests organized together. A collection contains a number of folders and HTTP requests.
- Inside the collection, we can create any number of requests as per our requirement. (Requests are a kind of file we can create to perform HTTP requests.)
- We can include pre-request and test scripts, use environment variables, and share collections with teams for collaboration.
- We can create, edit, delete, and run the collection.
- We can also create duplicate collections, and we can export it.
Major Requests we are going to use in the collection:
- GET – Retrieve the source from the data.
- POST – Create resource on database.
- PUT – Update existing resource on database.
- Patch – Update partial details of resource.
Delete – Delete existing resource from the database.
Understanding HTTP Status Codes
A status code is a three-digit number sent by a server in response to an HTTP request. It indicates the result of the request and whether it was successful, encountered an error, or requires further action.
200 Series:
200 – OK, 201 – Created, 202 – Accepted, 203 – Non-authoritative info, 204 – No content
400 Series:
400 – Bad request, 401 – Unauthorized, 403 – Forbidden, 404 – Not found, 409 – Conflict
500 Series:
500 – Internal server error, 501 – Not implemented, 502 – Bad gateway, 504 – Gateway timeout, 599 – Network timeout
Setting Up the Environment for API Testing
Pre-Requisites:
1.) NodeJS installation for creating a custom API
- INSTALL NodeJS from its official website.
- npm – Node Package Manager also comes with NodeJS.
- After installation, go to environment variables on your PC and check whether the path is configured or not.
- Also, run
node --version
in the CMD prompt to ensure its installation.
2.) Installing JSON Server
- Run
npm install -g json-server
in CMD.
- Create one JSON file with some JSON data in it. (This will be our custom API)
- Run that file in CMD as
json-server filename
.
Then our custom API will run on localhost (you can see the port in CMD), and we can perform HTTP requests via Postman.
What is JSON ?
JSON is expanded as JavaScript Object Notation.
- It is a lightweight, text-based format used to represent structured data. It is commonly used for transmitting data between a server and a client in web applications, especially in APIs.
- Basically, it was designed for human-readable data interchange. It is text, written with JavaScript notation.
- It is extended from the JS language. Extension is .json
- Its internet type (MIME) is application/json.
Terminologies:
- Strings should be written in double quotes and must have curly braces.
{"name":"jhon"}
- For number data, we don’t need to enclose it in double quotes.
{"age":30}
- If we want to give two values for one key, then we have to separate them with a comma (it is an array). →
{"phone":[123,456]}
- Boolean data does not require quotations. →
{"boolean":false}
.null
also doesn’t require quotations. - Arrays may store primitives →
{"data":[1,2,3]}
. And arrays having objects mean having all the formats like number and string.
Post Response Use Cases:
This section contains basic information and use cases about what we need to validate and know for Manual API Testing.
All the use cases and scripts are going to be executed in the Post-response tab in the ‘Scripts’ option.
Things we need to validate:-
- Status Code
- Headers
- Cookies
- Response Time
- Response Body
Initial Level Validations and Use Cases:
Take this URL as an example: https://reqres.in//api/users?page=2
‘’https://reqres.in’’ –> Host or Domain
‘’api/users?’’ –> Path Parameters
‘’page=2’’ –> Query Parameters (after the question mark is known as a query parameter)
‘’2’’ –> Resource
In Postman, while sending requests in JSON format (inside the collection), we need to select the ‘Raw’ option under the Body section.
pm – It is a Postman library that we are going to use to write scripts.
To write a test script in Postman, we have two common functions: Normal Function and Arrow Function.
Normal Function Syntax:
javascriptCopyEditpm.test("Test Name", function() {
// assertion;
});
Arrow Function Syntax:
javascriptCopyEditpm.test("Test Name", () => {
// assertion;
});
In the “Test Name” field, we can write our required texts related to the action we are going to perform.
In the “assertion” field, we are going to write the actual script.
We will use this syntax in the Postman Scripts option while using HTTP requests in a collection.
1.Testing the single status code :-
javascriptCopyEditpm.test("status code is 200", () => {
pm.response.to.have.status(200);
});
This syntax will test whether the HTTP response will return 200 as the status code or not.
We can change the status code as per our requirement in place of ‘200’.
2.Testing more than one status code :-
javascriptCopyEditpm.test("successful post request", () => {
pm.expect(pm.response.code).to.be.oneOf([200, 201]);
});
This syntax will test whether the HTTP response will return either 200 or 201.
3.Testing Status Code Text :-
javascriptCopyEditpm.test("status code is 200", () => {
pm.response.to.have.status("created");
});
It will check if the text of the status code is ‘created’ or not.
The post Manual API Testing with Postman – A Beginner-Friendly Guide appeared first on Spritle software.